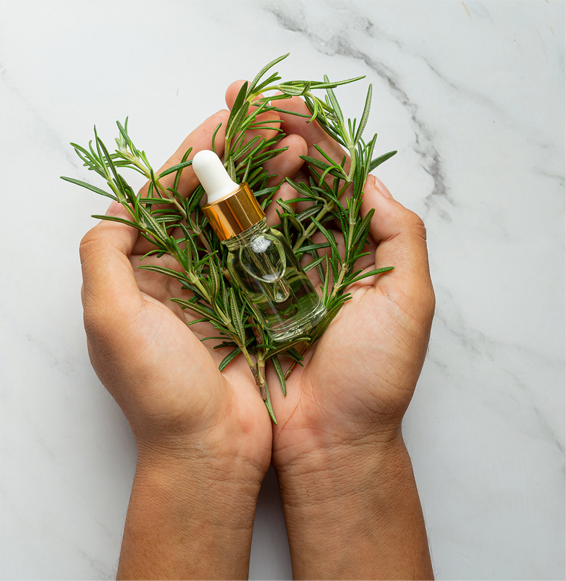

أفضل المنتجات ذات الجودة العالية
أسرار الطبيعة
متجر اسرار الطبيعة منتجات طبيعية للصحة والجمال جميع انواع العلاج بالأعشاب
آراء العملاء

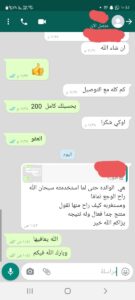

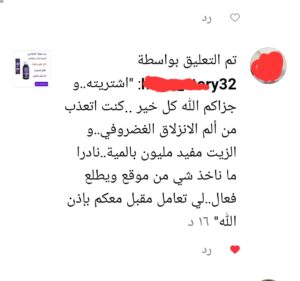
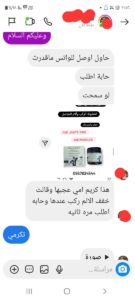
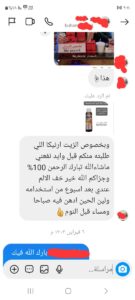
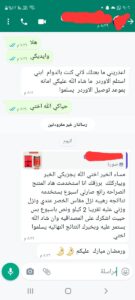
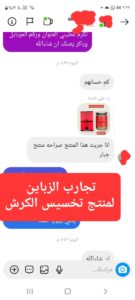

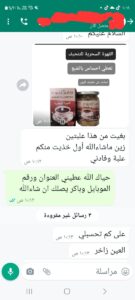

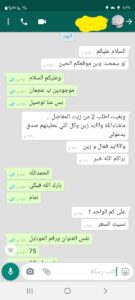
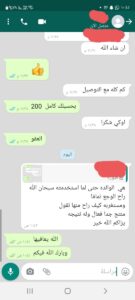
توصيل مجاني
أكثر من 500 درهم
توصيل لكافة دول الخليج
رسوم بسيطة لتوصيل لكافة دول الخليج
ضمان 100%
منتجاتنا ذات جودة عالية وقوية
ضمان استبدال المنتج
في حال عدم الرضا عن المنتج يمكنك استبداله